Unlocking Creative Control: Programmatic Color Handling in SwiftUI
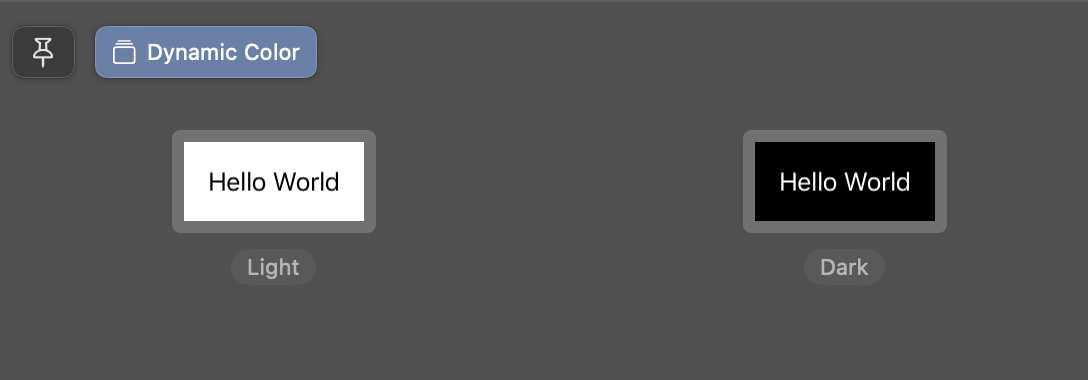
In the dynamic landscape of app design, colors play a pivotal role in shaping user experiences. While conventional color resources in Xcode offer a palette of choices, there's a captivating realm of creativity and control available through programmatic color handling in SwiftUI. This approach empowers developers to embrace individuality and customization, steering away from the constraints of predefined color resources.
Embracing Programmatic Freedom
When it comes to designing visually striking user interfaces, the ability to adapt and tailor colors on the fly becomes a powerful asset. By opting for programmatic color handling, developers can enjoy a level of creative control that enables them to define, blend, and transition colors according to their app's unique identity.
The Color Extension Magic
The foundation of programmatic color handling lies in extending the capabilities of SwiftUI's Color type. Let's explore two exciting extensions that can elevate your color handling game:
Dynamic Color Handling:
A world where colors adapt to the user's interface style. Imagine seamlessly transitioning from light to dark mode without a hitch. The dynamicColor function does just that. Whether you're on macOS or iOS, this function lets you define a color that automatically adapts based on the user's preference.
The magic of hex color codes now seamlessly blends with your SwiftUI views. By extending Color with a hex initializer, you can define colors using their hex representation, giving you pixel-perfect control over your color schemes.
import SwiftUI
import UIKit
extension Color {
static func dynamicColor(light: Color, dark: Color) -> Color {
#if os(macOS)
return Color(NSColor(dynamicProvider: { appearance in
appearance.name == .darkAqua ? dark : light
}))
#else
return Color(UIColor { (traitCollection: UITraitCollection) -> UIColor in
traitCollection.userInterfaceStyle == .dark ? dark.uiColor : light.uiColor
})
#endif
}
/// Initialise via hex value
init(hex: UInt, alpha: Double = 1.0) {
let red = Double((hex >> 16) & 0xFF) / 255.0
let green = Double((hex >> 8) & 0xFF) / 255.0
let blue = Double(hex & 0xFF) / 255.0
self.init(.sRGB, red: red, green: green, blue: blue, opacity: alpha)
}
/// Just a helper to get the uiColor for the UITraitCollection
private var uiColor: UIColor {
guard let cgColor else { fatalError("UNable to get cgcolor") }
return UIColor(cgColor: cgColor)
}
}
Bringing Color to Life
The dynamicColor function harmonizes your color scheme with the user's interface style. Apply it to your SwiftUI views effortlessly, and watch your app adapt to changing contexts without missing a beat.
struct DynamicColor_Previews: PreviewProvider {
static var previews: some View {
Button("Hello World") {}
.tint(.dynamicColor(light: .black, dark: .white))
.padding()
.previewLayout(.sizeThatFits)
}
}
Effortlessly create colors using their hex representation, merging design intuition with technical precision.
struct DynamicColor_Previews: PreviewProvider {
static var previews: some View {
Button("Hello World") {}
.tint(.dynamicColor(light: .init(hex: 0x000000), dark: .init(hex: 0xffffff)))
.padding()
.previewLayout(.sizeThatFits)
}
}
When it comes to color handling, SwiftUI's Color type forms the cornerstone. However, introducing custom extensions allows you to create a more descriptive and efficient way to manage your color palette. Let's dive into an extension that adds depth and clarity to your color definitions:
extension Color {
static let primary: Color = .dynamicColor(light: .black, dark: .white)
}
struct DynamicColor_Previews: PreviewProvider {
static var previews: some View {
Button("Hello World") {}
.tint(.primary)
.padding()
.previewLayout(.sizeThatFits)
}
}
Elevate Your Design Language
Programmatic color handling in SwiftUI is a journey of creative empowerment. It allows developers to infuse their apps with dynamic color transitions and to fine-tune color expressions to perfection. Whether you're crafting a seamless user experience or tailoring your app's visual identity, embracing programmatic color handling can be the key to unlocking your app's full potential. Step into this world of vibrant imagination and let your app's colors speak your language.